Form Submission API
The Form Submission API allows FormSmarts customers to programmatically submit a form.
The Form Submission API
To submit a form programmatically1, insert the form's ID into the URL and submit a POST
request:
API Endpoint | ||
---|---|---|
https://formsmarts.com/api/v1/forms/Form_ID /entries | ||
HTTP Method | ||
POST | ||
Parameter | Description | Notes |
field_id1, field_id2,… | The name of the parameter to set the value of each input field of a form is the field's numeric ID. See next section. | Optional for input fields which aren't mandatory on the form. |
context | Equivalent to the fs_ctxval form context parameter. | Optional Only allows alphanumeric characters and spaces. |
https://formsmarts.com/form/xyz
, its Form ID is xyz
.API Response
If the request is successful, the API returns an HTTP 200 response and a JSON object with the form entry's Reference Number:
{"reference_number": "DLNR3QPL2BZDZVKM37NSQF09X"}
If the request fails, the API returns a non-success HTTP status with a JSON object indicating the error:
{ "message": "Invalid parameter: 510289, 510290.", "error_code": "invalid_param", "params": ["510289", "510290"] }
Note: In some (rare) cases like if a server error occurs, the response body may contain plain text instead of JSON.
Implementation Note: Form Data Validation
When a user submits a form, FormSmarts validates their input and asks them to modify their entry if they provide invalid information.
Forms submitted via the API can only be accepted or rejected. If the data submitted is invalid, FormSmarts returns a HTTP 400 Bad Request error with a message, error code and a list of invalid parameters as illustrated above.
To reduce the chances of validation errors preventing successful requests:
- Implement strong validation on the data you submit
- Use general data types (e.g. Any Text instead of Letters Only) for general text input fields like people or company names
- Do not use the general Any Text data type for structured data like email, phone, or URLs. Use the specific Email, Phone or URL type instead
- Log all errors, i.e. any response with an HTTP status code different from 200.
Limitations
The following limitations apply to the Form Submission API:
- E-Signatures are not supported: you can submit a form that has an eSignature field, but the field must be Optional and not have a value.
Finding Input Field IDs
To find the ID of input fields of a form, log in to the API Console and submit a GET
request to the API endpoint https://formsmarts.com/api/v1/forms/Form_ID
/fields, as described in the Form API documentation.
For example, the screenshot below shows the IDs (right-most column) of the fields of the demo form used in the code example in the next section.
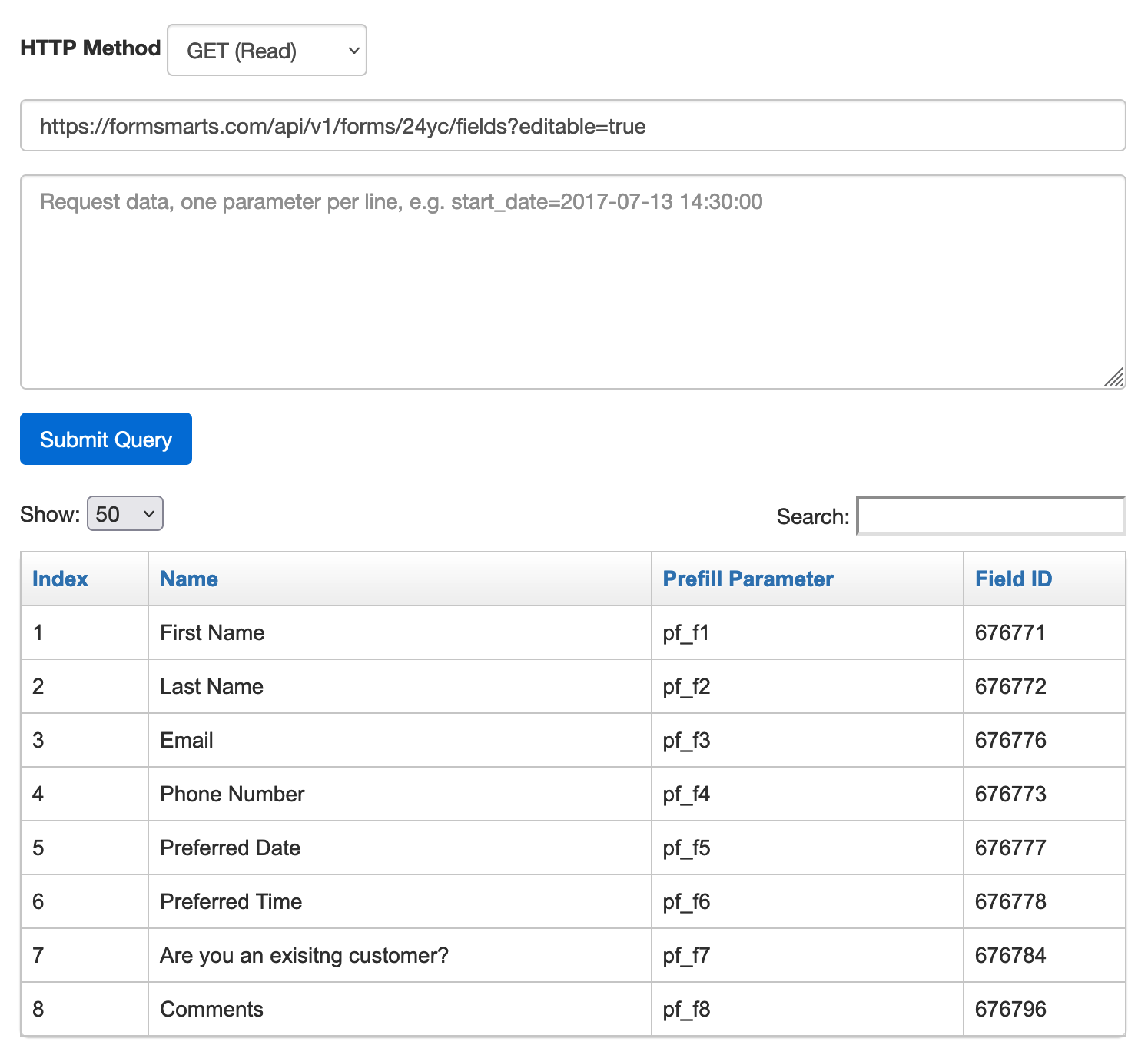
Authentication
FormSmarts verifies API requests with a JWT token in the Authorization header. You can sign requests with the FormSmarts API Client or a JWT library in your favorite programming language. You'll need to know your FormSmarts Account ID and secret FormSmarts API Key.
You'll find your Account ID in the Account Overview section of your account and your API Key in the Security Settings.
Python Example
Let's wrap this up with an example showing how to submit a form in Python.
#!/usr/bin/env python3 import json import requests import config from formsmarts_api import APIAuthenticator FORM_ID = '24yc' API_URL = 'https://formsmarts.com/api/v1/forms/{}/entries'.format(FORM_ID) # Values of input fields of demo form https://f8s.co/24yc FIELDS = { 676771: 'Jane', 676772: 'Doe', 676776: 'blackhole@syronex.com', 676773: '+1 (999) 999-9999', 676777: '2021-08-23', 676778: '15:35:30', 676784: 'No, this is my first appointment', 676796: '', # This fields is optional, we can either submit and empty value or leave the field out } class APITest: def __init__(self): self._au = APIAuthenticator(config.FORMSMARTS_ACCOUNT_ID, config.FORMSMARTS_API_KEY) def get_headers(self): return {APIAuthenticator.AUTH_HEADER: self._au.get_authorization_header()} def send_api_request(self, fields): resp = requests.post(API_URL, data=fields, headers=self.get_headers()) try: text = json.loads(resp.text) # Response should be JSON except json.JSONDecodeError: text = resp.text if resp.status_code == 200: print('Reference Number of the form entry: {}'.format(text['reference_number'])) else: print('Error {}: {}'.format(resp.status_code, resp.text)) if __name__ == '__main__': ApiTest().send_api_request(FIELDS)
The code above imports your Account ID and secret API Key form a config file, a Python module called config.py:
# You'll find your Account ID on: https://formsmarts.com/account/view FORMSMARTS_ACCOUNT_ID = 'FSA-999999' # You'll find your API Key on: https://formsmarts.com/account/view#security-settings FORMSMARTS_API_KEY = 'TqE35BBzxfmxC74YQ4jQCPFx1oKvFhECOfWrbTh8fVMG6viZWiTfvh4dOZSSK71v'
Node.js Example
Here is another code example in Node.js.
The only functional difference with the code above is that the Account ID and secret API Key are now defined in the environment variables FORMSMARTS_ACCOUNT_ID
and FORMSMARTS_API_KEY
respectively.
const crypto = require('crypto') const OAuth = require('oauth-1.0a') const got = require('got') const FormData = require('form-data') const FORM_ID = '24yc'; const API_URL = `https://formsmarts.com/api/v1/forms/${FORM_ID}/entries`; // Values of input fields of demo form https://f8s.co/24yc const FIELDS = { 676771: 'Jane', 676772: 'Doe', 676776: 'blackhole@syronex.com', 676773: '+1 (999) 999-9999', 676777: '2021-08-23', 676778: '15:35:30', 676784: 'No, this is my first appointment', 676796: '', } function authHeader(fields){ const oauth = OAuth({ consumer: { // Get consumer key and secret from environment variables key: process.env.FORMSMARTS_ACCOUNT_ID, secret: process.env.FORMSMARTS_API_KEY, }, signature_method: 'HMAC-SHA1', hash_function(base_string, key) { return crypto.createHmac('sha1', key).update(base_string).digest('base64'); }, }); return oauth.toHeader(oauth.authorize({url: API_URL, method: 'POST', data: fields})); } function sendApiRequest(fields){ (async () => { try { const resp = await got.post(API_URL, { body: formData(fields), responseType: 'json', headers: authHeader(fields) }); console.log('Reference Number of the form entry: ' + resp.body.reference_number); } catch (error) { console.log(`${error.response.statusCode} ${error.response.body.message}`); } })(); } function formData(object) { const formData = new FormData(); Object.keys(object).forEach(key => formData.append(key, object[key])); return formData; } sendApiRequest(FIELDS);
Uploading Form Attachments
To submit a form that allows the user to upload form attachments with the API, you first need to upload the files, then submit the form.
- Upload files: submit a
POST
request to the Upload API endpoint below for each upload field on the form - Submit the form: pass the Upload ID returned by the API as the value of the corresponding upload field.
The Upload API
To upload a form attachment, submit a POST
request:
API Endpoint | ||
---|---|---|
https://formsmarts.com/api/v1/attachments | ||
HTTP Method | ||
POST | ||
Parameter | Description | |
form_id | The ID of the form | |
File | Description | |
Field_ID | Use the ID of the upload field as the name of the form attachment. |
To download a form attachment, insert its Upload ID into the URL and submit a GET
request:
API Endpoint | ||
---|---|---|
https://formsmarts.com/api/v1/attachments/Upload_ID | ||
HTTP Method | ||
GET |
Example: Upload and Submit
The code below shows how to automatically submit a form and upload a picture with Python. The form we use for this example is this upload demo.
#!/usr/bin/env python3 import json import config import requests from formsmarts_api import APIAuthenticator FORM_ID = 'lqh' UPLOAD_FIELD_ID = 122621 UPLOAD_API_URL = 'https://formsmarts.com/api/v1/attachments' SUBMIT_API_URL = 'https://formsmarts.com/api/v1/forms/{}/entries'.format(FORM_ID) FILE_PATH = '/tmp/test-image.png' # Values of input fields of demo form https://f8s.co/lqh fields = { 122619: 'Jane Doe', 122620: 'blackhole@syronex.com', UPLOAD_FIELD_ID: '', # Fill with the Upload ID returned by the Upload API 123744: "Hello,\nThanks for playing.", } class APITest(): def __init__(self): self._au = APIAuthenticator(config.FORMSMARTS_ACCOUNT_ID, config.FORMSMARTS_API_KEY) def get_headers(self): return {APIAuthenticator.AUTH_HEADER: self._au.get_authorization_header()} def upload_attachment(self, endpoint, field_id, form_id, file_path): files = {str(field_id): (file_path.split('/')[-1], open(file_path, 'rb'), 'image/png')} resp = requests.post(url=endpoint, params=dict(form_id=form_id), files=files, headers=self.get_headers()) if resp.status_code == 200: resp = resp.json() print('Upload successful: {}'.format(resp)) return resp else: print('Error {}: {}'.format(resp.status_code, resp.text)) def submit_form(self, fields, uploads): # Set upload field value to the Upload ID returned by the Upload API fields[UPLOAD_FIELD_ID] = uploads['files'][0]['id'] resp = requests.post(SUBMIT_API_URL, data=fields, headers=self.get_headers()) if resp.status_code == 200: text = json.loads(resp.text) print('Reference Number of the form response: {}'.format(text['reference_number'])) else: print('Error {}: {}'.format(resp.status_code, resp.text)) if __name__ == '__main__': test = APITest() uploads = test.upload_attachment(UPLOAD_API_URL, UPLOAD_FIELD_ID, FORM_ID, FILE_PATH) test.submit_form(fields, uploads)
- Not available with Business Starter and Plus accounts.
FormSmarts API Client
- FormSmarts API Client (Python)
- FormSmarts API Client (Node.js)
Need Help?
- Send API questions to @FormSmartsTech